Introduction
Inngest is an open source platform that adds superpowers to serverless functions.
Using our SDK, a single line of code adds retries, queues, sleeps, cron schedules, fan-out jobs, and reliable steps to serverless functions in your existing projects. It's deployable to any platform, without any infrastructure or configuration. And, everything is locally testable via our UI.
Learn how to get started in our quick start tutorial, or continue reading for an example.
A small but powerful example
Adding sleeps, retries, and reliable steps to a function:
import { createStepFunction } from "inngest";
type UserSignup = {
name: "user/new.signup",
data: {
email: string;
name: string;
}
}
export default createStepFunction<UserSignup>("Signup flow", "user/new.signup", ({ event, tools }) => {
// If this step fails it will retry automatically. It will only
// run once if it succeeds ⚡
const promo = tools.run("Generate promo code", async () => {
const promoCode = await generatePromoCode();
return promoCode.code;
});
// Again, if the email provider is down this will retry - but we will only
// generate one promo code ⚡
tools.run("Send a welcome promo", async () => {
await sendEmail({ email: event.data, promo });
});
// You can sleep on any platform! 😴
tools.sleep("1 day");
// This runs exactly 1 day after the user signs up ⏰
tools.run("Send drip campaign", async () => {
await sendDripCampaign();
});
})
In this example, you can reliably run serverless functions even if external APIs are down. You can also sleep or delay work without configuring queues. Plus, all events, jobs, and functions are strictly typed via TypeScript for maximum correctness. Here's how things look when you locally run:
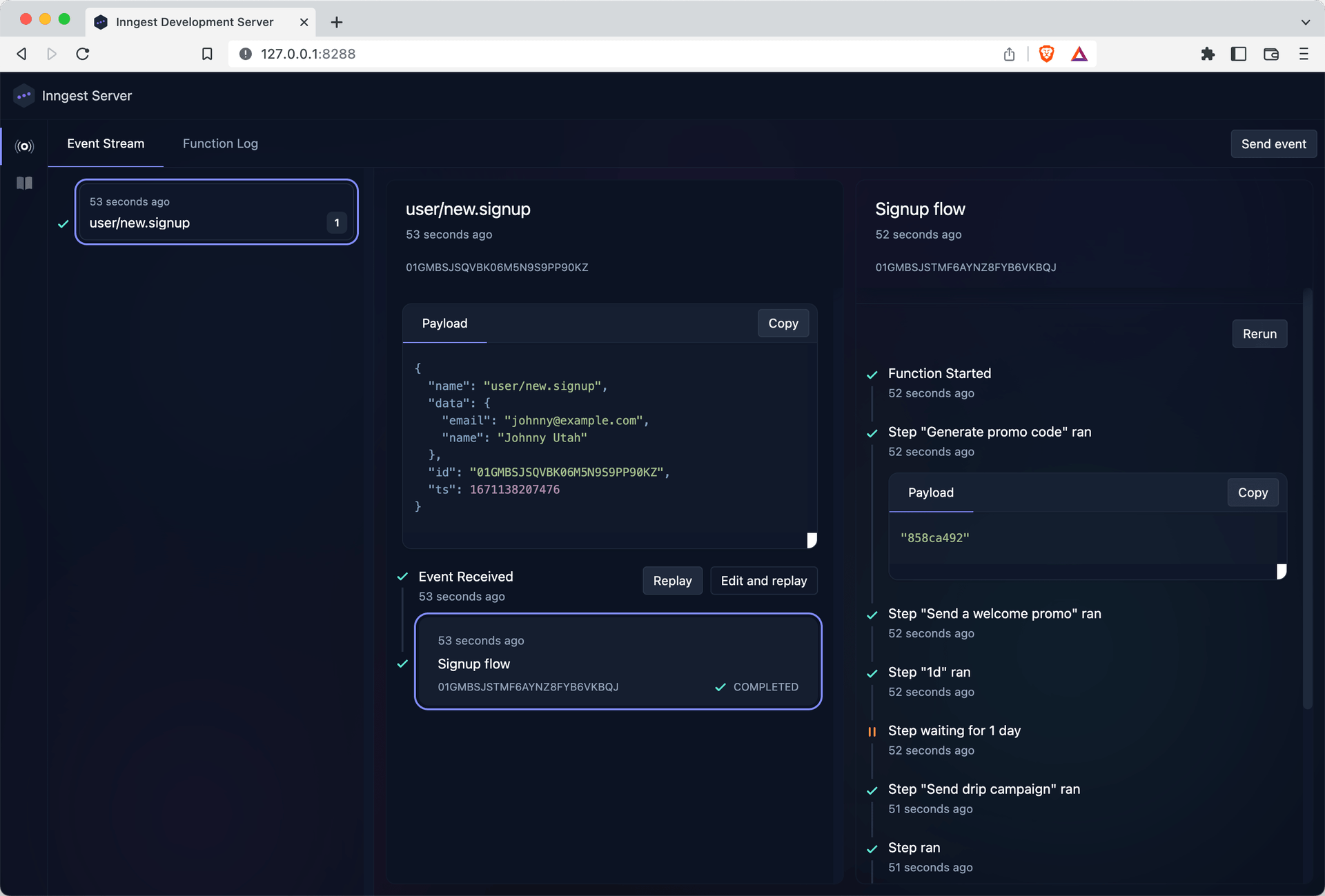
Comparisons
Without Inngest, you would have to configure several jobs amongst several different queues, then handle retries yourself. There's also a chance that many promo codes are generated depending on the reliability of that API. With Inngest, you can push this function live and everything happens automatically.
Getting started
From here, you might want to:
- Get started via our quick-start tutorial
- Learn more about functions and the tools provided
- Learn how to integrate with your platform of choice
Resources and help
If you have any questions we're always around in our Discord community or on our GitHub.